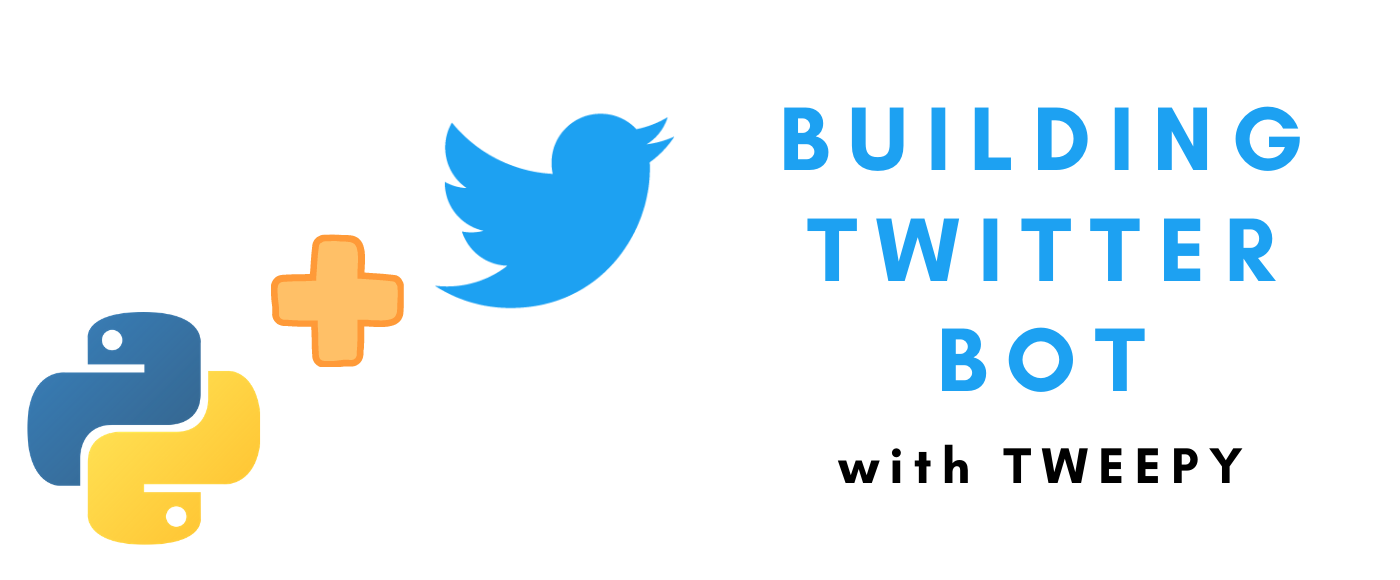
Build a Twitter Bot With Tweepy(Source Code Included)
As we all know, Twitter is the most famous social media in the world. Because it is based on social networking of strangers(compared to Facebook, which belongs to acquaintance social media), we can obtain a large amount of traffic from it easily. How to become popular on Twitter, how to get accurate traffic through Twitter, and how to quickly build your own Twitter marketing system.
This article will introduce Tweepy, a Python-based Twitter library, and teach you step by step how to build your own Twitter Bot with Tweepy. The most important thing is: the source code is included.
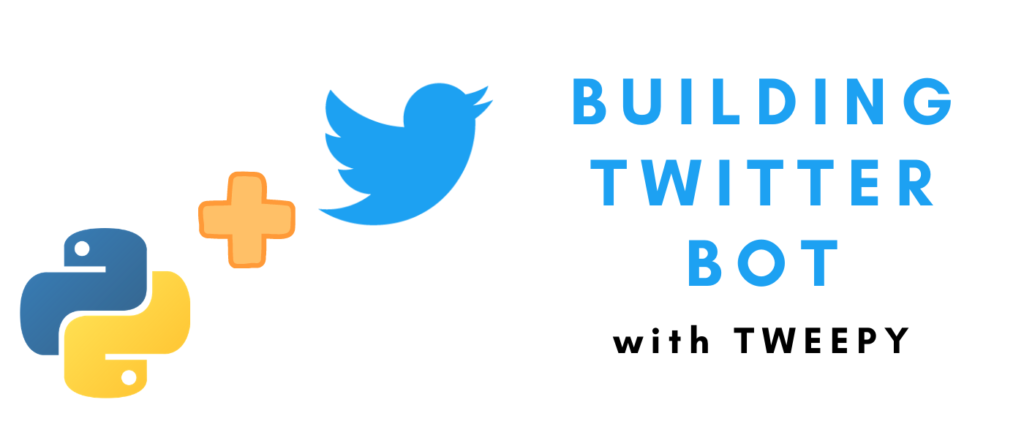
In “Twitter Automation Tools & Growing Strategies“, we introduced Twitter automation tools and Twitter account growing strategy, but these tools are neither free nor meet our needs. On the other hand, Twitter Media Studio, which we introduced in “Powerful official Twitter Posting Tool – Media Studio“, also needs us to set the post time and content of each tweet accurately, which is a nightmare when growing multiple accounts.
So we will use Tweepy to build our own Twitter Bot, which supports Twitter regular operations, and is truly Set & Forget. Of course, you can also use the paid tools recommended in the previous article if you feel troublesome to build your own bot.
Features that Twitter Bot must have
- Auto post tweets: post tweets with text, images, and videos, set the posting interval, select images and videos from the specified directory automatically for posting.
- Account search: fetch the followings and followers list of a specified account, multiple filtering conditions supported(number of followings, number of followers, activity, etc.).
- Automatic follow: Automatic follow accounts, set the number limit.
- Automatic unfollow: For accounts not follow back, automatic unfollow after N days, the number of unfollows per day can be configured.
- Automatic retweet: search tweets by keyword, automatic retweet, and the number of retweet per day can be configured.
As you can see, our Twitter Bot integrates operations such as posting, following, and retweeting. As long as it is set up, it can automatically grow accounts. If we have multiple Twitter accounts, we can easily build a Twitter marketing system.
In addition, we use the best Twitter library based on Python – Tweepy, which can be downloaded from GitHub. It is compatible with Window, Linux and other systems. Our Twitter Bot will be running on a VPS, so we will use CentOS 8 + Python 3 + Tweepy as an example. Now, let’s start.
Prepare a VPS
In the first step, we need a VPS to run Twitter Bot, it needs to be able to access Twitter (so it can’t be a machine in China), it needs to be fast enough, it needs to be cheap enough, and it needs to be able to easily replace the IP. We highly recommend Vultr VPS.
For the process of installing CentOS 8, please refer to this article “How to install ShadowSocksR(SSR) server in CentOS 7 – Complete Tutorial(2019)“, here we assume that you have completed the installation of CentOS 8.
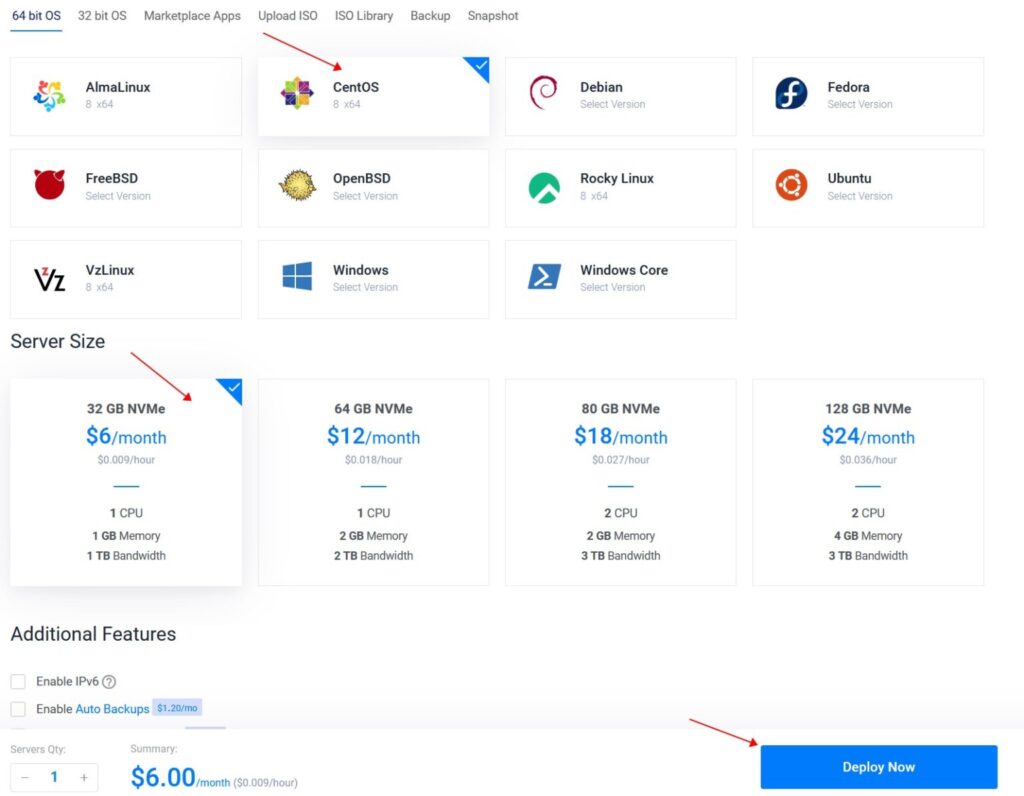
pip3 and python3 installation
The second step, check whether pip3 and python3 have been installed.
Run these commands:
pip3 -V
python3 -V

You can see that the pip version installed is 9.0.3, and the python version is 3.6.8, which can meet the requirements. If your pip version and python version are low, you can use the following command to upgrade:
yum install -y python3-pip
Install Tweepy
The third step is to install Tweepy.
Run the following commands:
pip3 install tweepy
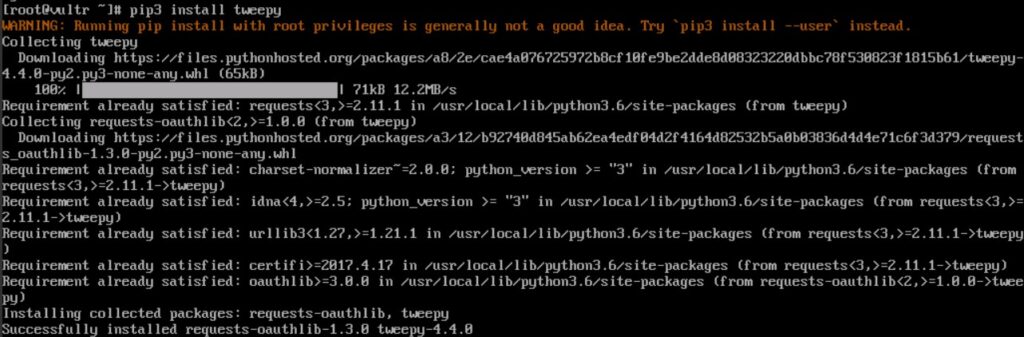
OK, we have installed Tweepy successfully.
Get the keys of your Twitter account
The fourth step, get the keys to access & update your Twitter account, which mainly includes:
- Consumer key
- Consumer secret
- Access token
- Access secret
The functions of the keys are described in Twitter’s official Developer document, so we won’t discuss it here. You only need to know that you need these four keys to connect to your Twitter account, post Tweets, and follow accounts.
So where can you find the keys?
- Visit Twitter developer homepage (https://developer.twitter.com), click Sign Up, and Twitter will ask you a series of questions, such as what does your program do? Is it for government? What is your purpose of applying for a developer account? After the request is completed, Twitter will review it. The review process is very fast, just wait for a while.
- Go back to the developer homepage, click Apps, then select Create an app, and fill in the app’s name, icon, url, etc. In Keys and Tokens, you will find the API Key, API secret key, corresponding to Consumer key and Consumer secret, and below is the Access token and Access token secret. Please make sure that the Access level is Read, write, and Direct Messages, if not, go to the Permissions tab to modify.
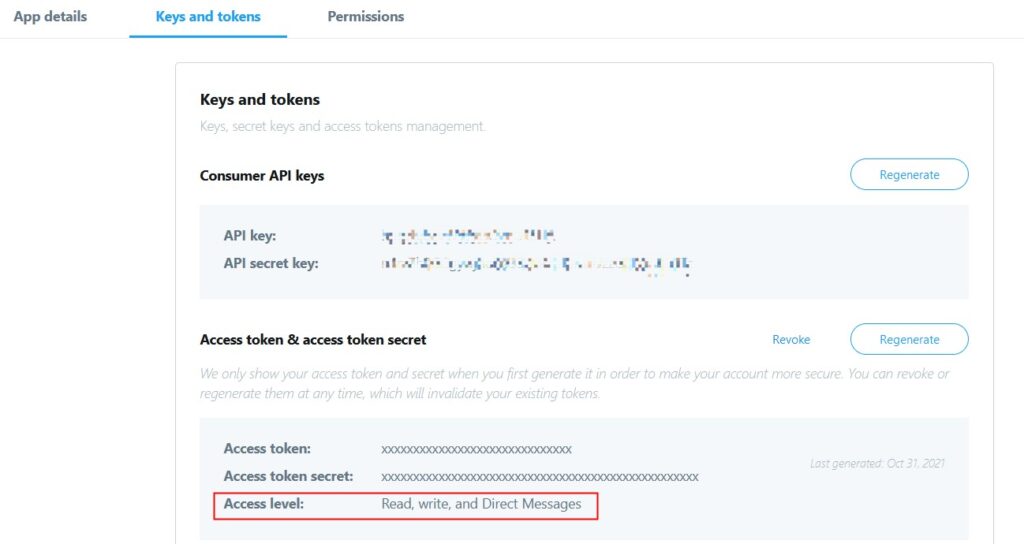
Note: Please keep the keys of your account very carefully. With these four keys, anyone can control your twitter account.
Build the bot with Python + Tweepy
After the four steps above, we finally have everything prepared, now the fifth step, also the final step, use Python and Tweepy to build the bot.
- Test whether your account is correctly connected to twitter.
The Python code is given below.
import tweepy
# Authenticate to Twitter
auth = tweepy.OAuthHandler("your-consumer-key", "your-consumer-secret")
auth.set_access_token("your-access-token", "your-access-secret")
api = tweepy.API(auth)
try:
api.verify_credentials()
print("Authentication OK")
except:
print("Error during authentication")
Run the script.

The authentication is successful.
- Post a text tweet, the content is the classical “Hello World!”.
import tweepy
# Authenticate to Twitter
auth = tweepy.OAuthHandler("your-consumer-key", "your-consumer-secret")
auth.set_access_token("your-access-token", "your-access-secret")
# Create API object
api = tweepy.API(auth)
# Create a tweet
api.update_status("Hello World!")
- Scan the videos in a specified directory, post a video every specified time (for example, 1 hour), and move the sent file to another directory after the transmission is completed.
import tweepy
import os
import time
import shutil
source_dir = '/tweepy/video'
target_dir = '/tweepy/done/'
check_after_secs = 3600
tweet = ''
# Authenticate to Twitter
auth = tweepy.OAuthHandler("your-consumer-key", "your-consumer-secret")
auth.set_access_token("your-access-token", "your-access-secret")
# Create API object
api = tweepy.API(auth)
try:
for root, sub_dirs, files in os.walk(source_dir):
for special_file in files:
spcial_file_dir = os.path.join(root, special_file)
print('Processing video %s' % spcial_file_dir)
media = api.media_upload(filename=spcial_file_dir,media_category="tweet_video")
post_result = api.update_status(status=tweet,media_ids=[media.media_id])
# Move uploaded file
shutil.move(spcial_file_dir, target_dir)
print('Checking after %s seconds' % str(check_after_secs))
time.sleep(check_after_secs)
except:
print("Error during read dir")
Note: we must upload the video using the media_upload method of the api object, and the media_category must be set to “tweet_video“, then use the update_status method of the api object to send the tweet, and specify the media_ids as the media_id of the video just uploaded, the text of the tweet can be empty.
Summary
Well, the above is the whole process of using Tweepy to make a Twitter Bot, we have tested all the steps. For more content, please continue to follow us.
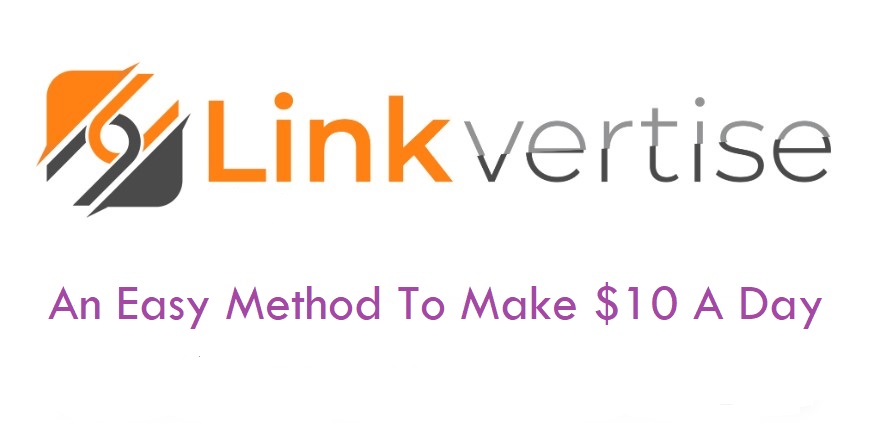
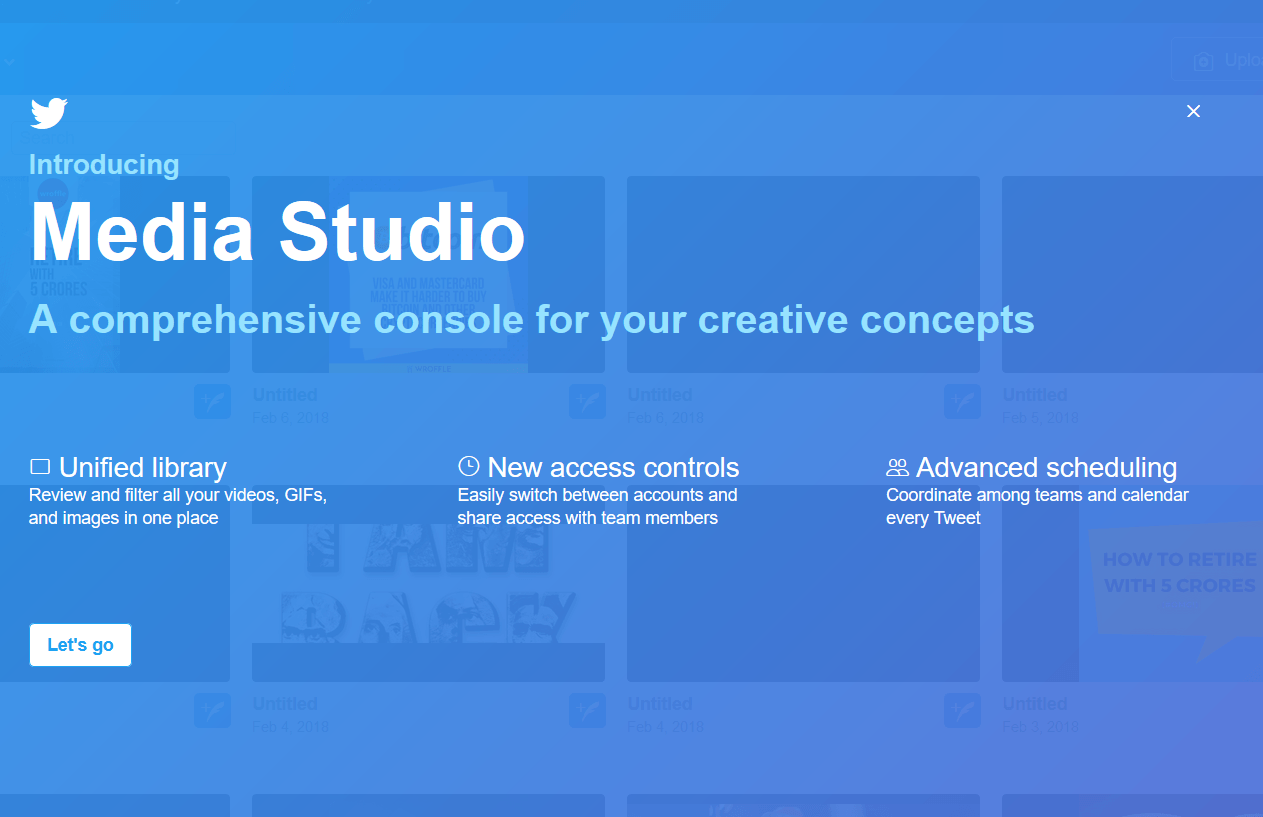